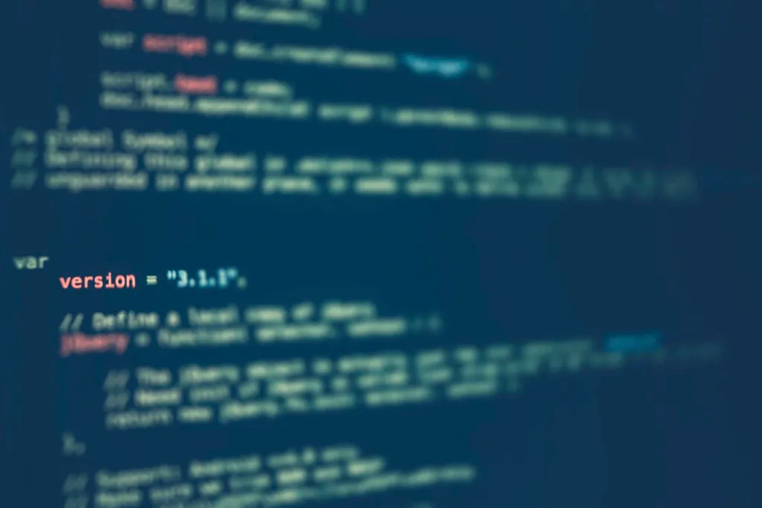
In this section, we will discuss SQL injections, a persistent issue that requires careful attention due to its serious implications. Let’s delve into this problem.
Imagine we have a query that retrieves the “city” value from a submitted HTML form, and we decide to insert this input directly into our SQL query like this:

Using dynamic SQL queries in this manner should be avoided, especially when the input comes from users. What could go wrong? If the user inputs a legitimate city name, such as “Dubai,” the resulting query will be correct: “SELECT * FROM Orders WHERE City = ‘Dubai’.” However, if a user enters something malicious like “Dubai’; DROP TABLE Orders –“, the query changes to “SELECT * FROM Orders WHERE City = ‘Dubai’; DROP TABLE Orders –“, which could cause significant damage. The double dashes signify a comment, allowing the harmful command to execute and potentially erase all Orders data.
It’s essential not to depend solely on client-side data. So, how can we mitigate this risk? Let’s explore a few solutions.
One approach is to use stored procedures:

In this example, I’ve used a SQL data adapter, though it’s not strictly necessary. Generally, stored procedures can offer a secure method, provided that dynamic SQL isn’t used within them. At the very least, this approach helps protect against user input.
Another option is to use parameterized queries, as shown here:

In this case, we use ADO.NET parameters to tackle SQL injection risks. This method effectively helps prevent such vulnerabilities.
Finally, employing an ORM (Object-Relational Mapping) framework like Entity Framework or NHibernate can provide built-in protection against SQL injections. However, it remains crucial to validate user inputs and be cautious about potentially dangerous operations.
By implementing these strategies, we can strengthen our defenses against SQL injections and ensure the integrity and security of our applications.
Source: Nguyen Chi Hieu – Technical Manager